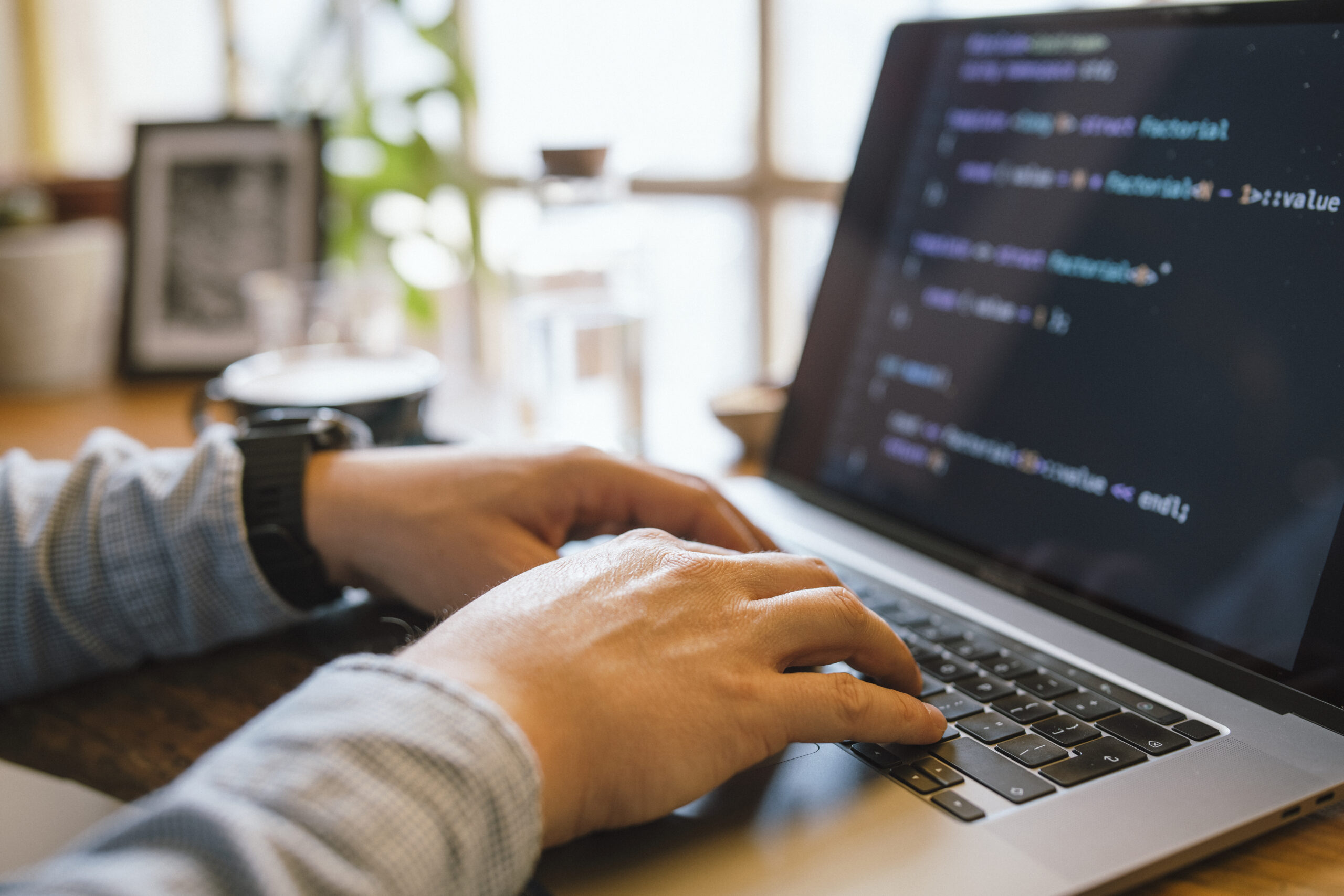
Debugging is Among the most essential — nevertheless generally forgotten — competencies in a developer’s toolkit. It is not almost repairing damaged code; it’s about comprehending how and why items go Mistaken, and Mastering to Assume methodically to unravel challenges efficiently. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can preserve hrs of irritation and radically help your efficiency. Here's various tactics to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Tools
One of the quickest ways builders can elevate their debugging capabilities is by mastering the resources they use each day. While creating code is one Element of progress, being aware of how you can connect with it effectively all through execution is equally essential. Fashionable growth environments arrive equipped with potent debugging abilities — but several builders only scratch the floor of what these tools can perform.
Just take, for instance, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools assist you to set breakpoints, inspect the value of variables at runtime, action via code line by line, and in many cases modify code within the fly. When made use of effectively, they Allow you to notice precisely how your code behaves through execution, that is priceless for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They help you inspect the DOM, keep track of network requests, check out serious-time efficiency metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can switch annoying UI issues into manageable duties.
For backend or process-level developers, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around operating processes and memory administration. Discovering these equipment could possibly have a steeper learning curve but pays off when debugging efficiency troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become at ease with Variation control methods like Git to comprehend code historical past, come across the precise moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications usually means likely past default options and shortcuts — it’s about building an intimate understanding of your growth setting making sure that when problems arise, you’re not lost at midnight. The better you realize your tools, the greater time you'll be able to devote solving the actual problem rather than fumbling through the process.
Reproduce the issue
Probably the most critical — and infrequently forgotten — techniques in powerful debugging is reproducing the situation. In advance of jumping in to the code or making guesses, builders need to have to create a steady atmosphere or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug gets a video game of likelihood, frequently bringing about wasted time and fragile code changes.
The first step in reproducing a dilemma is accumulating just as much context as you possibly can. Talk to inquiries like: What actions brought about the issue? Which ecosystem was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it results in being to isolate the precise situations under which the bug happens.
When you’ve gathered sufficient facts, endeavor to recreate the issue in your neighborhood environment. This might mean inputting precisely the same data, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, look at writing automated checks that replicate the edge conditions or state transitions concerned. These checks not only aid expose the condition and also reduce regressions Later on.
Often, The difficulty might be setting-unique — it would transpire only on sure operating techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It necessitates tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be previously midway to correcting it. With a reproducible scenario, you can use your debugging resources much more efficiently, check probable fixes properly, and converse additional Plainly with the staff or end users. It turns an abstract grievance into a concrete challenge — Which’s the place developers thrive.
Read and Realize the Error Messages
Error messages are often the most beneficial clues a developer has when a little something goes Erroneous. In lieu of observing them as discouraging interruptions, builders must discover to take care of mistake messages as direct communications in the system. They frequently show you just what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Begin by reading the concept very carefully As well as in entire. Several builders, particularly when beneath time stress, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and understand them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it issue to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also helpful to grasp the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and Studying to acknowledge these can significantly accelerate your debugging system.
Some mistakes are obscure or generic, As well as in those circumstances, it’s important to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date changes from the codebase.
Don’t overlook compiler or linter warnings either. These usually precede much larger challenges and provide hints about possible bugs.
Eventually, error messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a much more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most powerful tools inside a developer’s debugging toolkit. When utilized successfully, it provides true-time insights into how an software behaves, supporting you recognize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
A great logging approach begins with realizing what to log and at what degree. Typical logging levels include DEBUG, Facts, Alert, ERROR, and Deadly. Use DEBUG for thorough diagnostic details in the course of improvement, INFO for typical situations (like prosperous start off-ups), Alert for prospective problems that don’t crack the appliance, ERROR for actual complications, and Deadly once the program can’t carry on.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and slow down your method. Deal with key gatherings, state variations, enter/output values, and critical final decision factors in the code.
Format your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re In particular precious in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. By using a perfectly-imagined-out logging tactic, you are able to decrease the time it's going to take to spot challenges, acquire further visibility into your applications, and Enhance the In general maintainability and dependability within your code.
Think Like a Detective
Debugging is not only a complex endeavor—it is a method of investigation. To effectively recognize and correct bugs, builders will have to approach the process like a detective fixing a mystery. This attitude can help stop working elaborate issues into manageable elements and comply with clues logically to uncover the basis bring about.
Begin by gathering evidence. Look at the signs of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys a criminal offense scene, collect just as much applicable information as you can with out jumping to conclusions. Use logs, exam conditions, and person experiences to piece together a clear photograph of what’s going on.
Upcoming, kind hypotheses. Question oneself: What could possibly be leading to this behavior? Have any adjustments not too long ago been produced to the codebase? Has this difficulty happened ahead of below comparable circumstances? The intention will be to slender down opportunities and recognize possible culprits.
Then, exam your theories systematically. Endeavor to recreate the trouble inside a managed setting. In the event you suspect a particular functionality or part, isolate it and verify if The difficulty persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you nearer to the reality.
Pay out shut consideration to little facts. Bugs usually disguise while in the least predicted places—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be thorough and client, resisting the urge to patch the issue with no fully comprehension it. Short term fixes may perhaps conceal the actual problem, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging system can conserve time for foreseeable future issues and aid Some others comprehend your reasoning.
By contemplating similar to a detective, builders can sharpen their analytical abilities, technique issues methodically, and turn into more practical at uncovering concealed problems in sophisticated devices.
Generate Tests
Creating assessments is among the simplest strategies to transform your debugging skills and General growth effectiveness. Assessments don't just assistance catch bugs early but also serve as a safety Internet that provides you self esteem when building variations to your codebase. A well-tested application is much easier to debug mainly because it allows you to pinpoint specifically the place and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These small, isolated checks can immediately expose whether or not a specific bit of logic is Doing the job as predicted. Every time a examination fails, you right away know in which to appear, considerably reducing some time spent debugging. Device assessments are Specifically helpful for catching regression bugs—issues that reappear just after Earlier currently being set.
Next, combine integration exams and conclude-to-finish checks into your workflow. These enable be certain that numerous parts of your software perform together efficiently. They’re specifically useful for catching bugs that come about in elaborate techniques with numerous parts or providers interacting. If something breaks, your assessments can tell you which Component of the pipeline failed and underneath what situations.
Crafting assessments also forces you to Imagine critically about your code. To check a characteristic thoroughly, you will need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to raised code construction and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails consistently, you'll be able to center on fixing the bug and enjoy your test move when The problem is fixed. This method makes certain that the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable system—helping you catch far more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your display for several hours, trying Answer right after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks helps you reset your thoughts, minimize stress, and sometimes see The difficulty from the new standpoint.
If you're far too near the code for far too long, cognitive exhaustion sets in. You might start overlooking obvious mistakes or misreading code which you wrote just hrs earlier. In this particular condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee break, or perhaps switching to a different task for ten–quarter-hour can refresh your concentration. Quite a few developers report locating the root of a dilemma when they've taken time and energy to disconnect, allowing their subconscious function within the history.
Breaks also enable avert burnout, Specifically through for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping absent enables you to return with renewed energy and also a clearer attitude. You might quickly recognize a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to move all over, stretch, or do a thing unrelated to code. It might experience counterintuitive, Specially less than restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks just isn't a sign of weak point—it’s a wise strategy. It provides your Mind House to breathe, improves your viewpoint, and allows you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to improve as a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing important if you make an effort to mirror and examine what went Mistaken.
Start out by inquiring you a few important queries after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with superior techniques like device tests, code assessments, or logging? The responses normally expose blind places with your workflow or knowledge and make it easier to Make more robust coding practices relocating forward.
Documenting bugs may also be a great behavior. Keep a developer journal or maintain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent problems—which you can proactively stay away from.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Primarily highly effective. No matter whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Some others stay away from the same challenge boosts crew efficiency and cultivates a more robust Studying society.
Far more importantly, viewing bugs as classes shifts your frame of mind from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as crucial parts of your growth journey. In the end, many of the greatest builders usually are not those who create great code, but those that repeatedly discover from their problems.
In the end, Every single bug you fix adds a new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Bettering your debugging competencies requires time, follow, and tolerance — but the payoff is huge. It would make you a far more efficient, self-confident, and able developer. Another time you're knee-deep within a mysterious read more bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.